Build. Monetize. Scale.
Build. Monetize. Scale.
Build. Monetize. Scale.
Build onchain apps, games, and agents with reliable wallet infrastructure, practical dev tools & in-depth onchain data: for all builders, on every EVM.
Build onchain apps, games, and agents with reliable wallet infrastructure, practical dev tools & in-depth onchain data: for all builders, on every EVM.
Build onchain apps, games, and agents with reliable wallet infrastructure, practical dev tools & in-depth onchain data: for all builders, on every EVM.
TRANSACTIONS
CONTRACTS
WALLETS
TRANSACTIONS
CONTRACTS
WALLETS
TRANSACTIONS
CONTRACTS
WALLETS
See thirdweb in action
See thirdweb in action
See thirdweb in action
Nebula
Most powerful AI for blockchain
Read real-time and historical blockchain data, write to any blockchain in natural language with powerful reasoning capabilities.
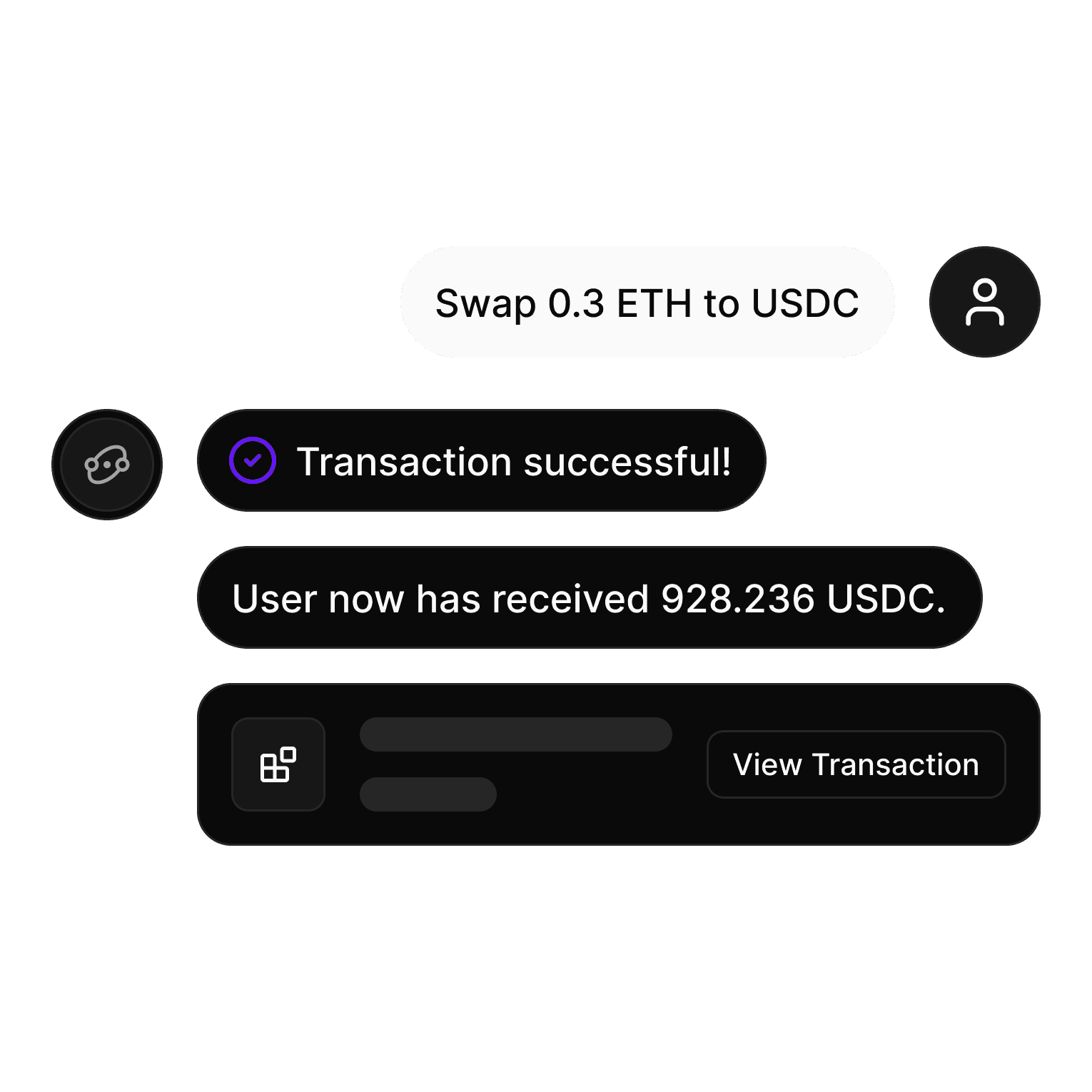
Nebula
Most powerful AI for blockchain
Read real-time and historical blockchain data, write to any blockchain in natural language with powerful reasoning capabilities.
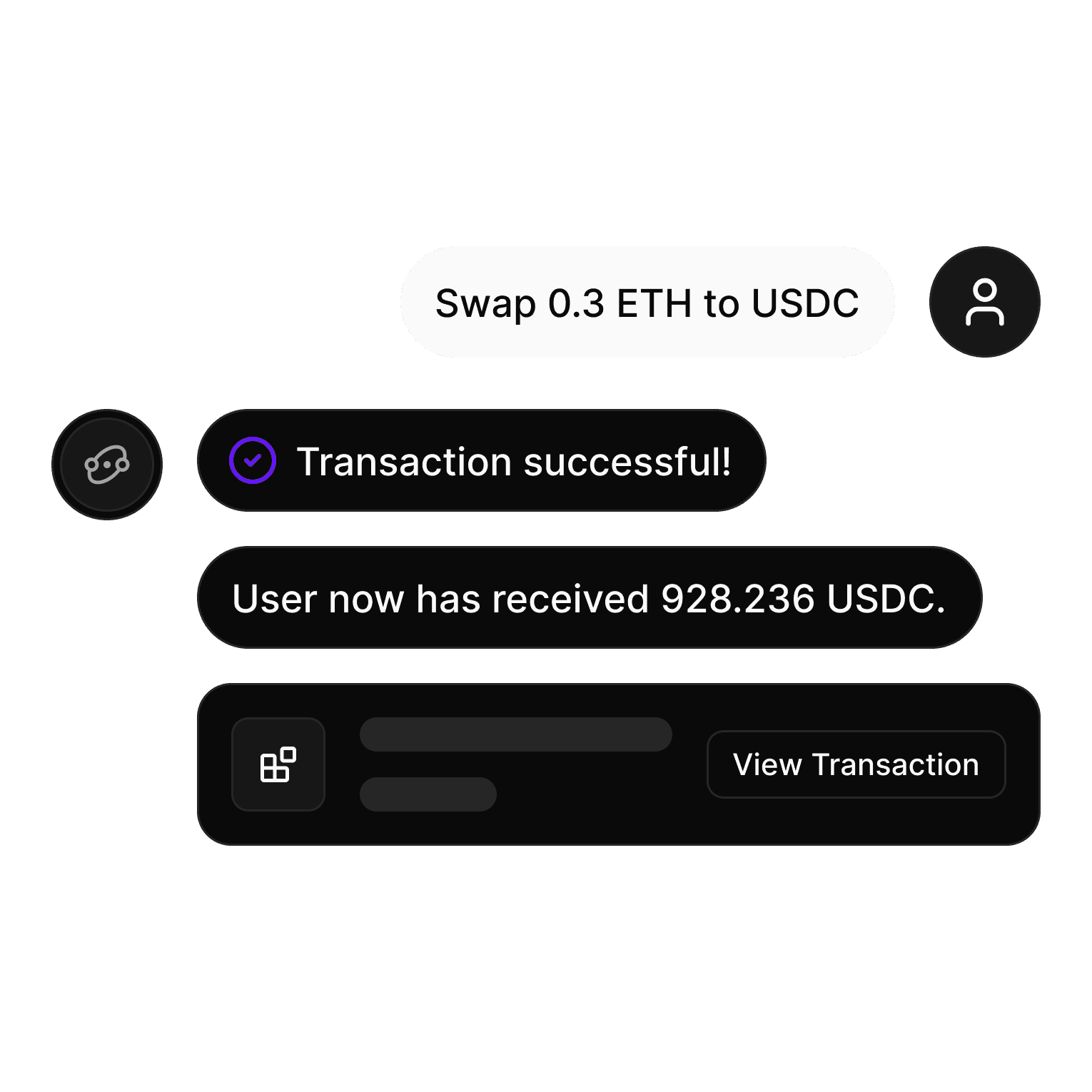
Nebula
Most powerful AI for blockchain
Read real-time and historical blockchain data, write to any blockchain in natural language with powerful reasoning capabilities.
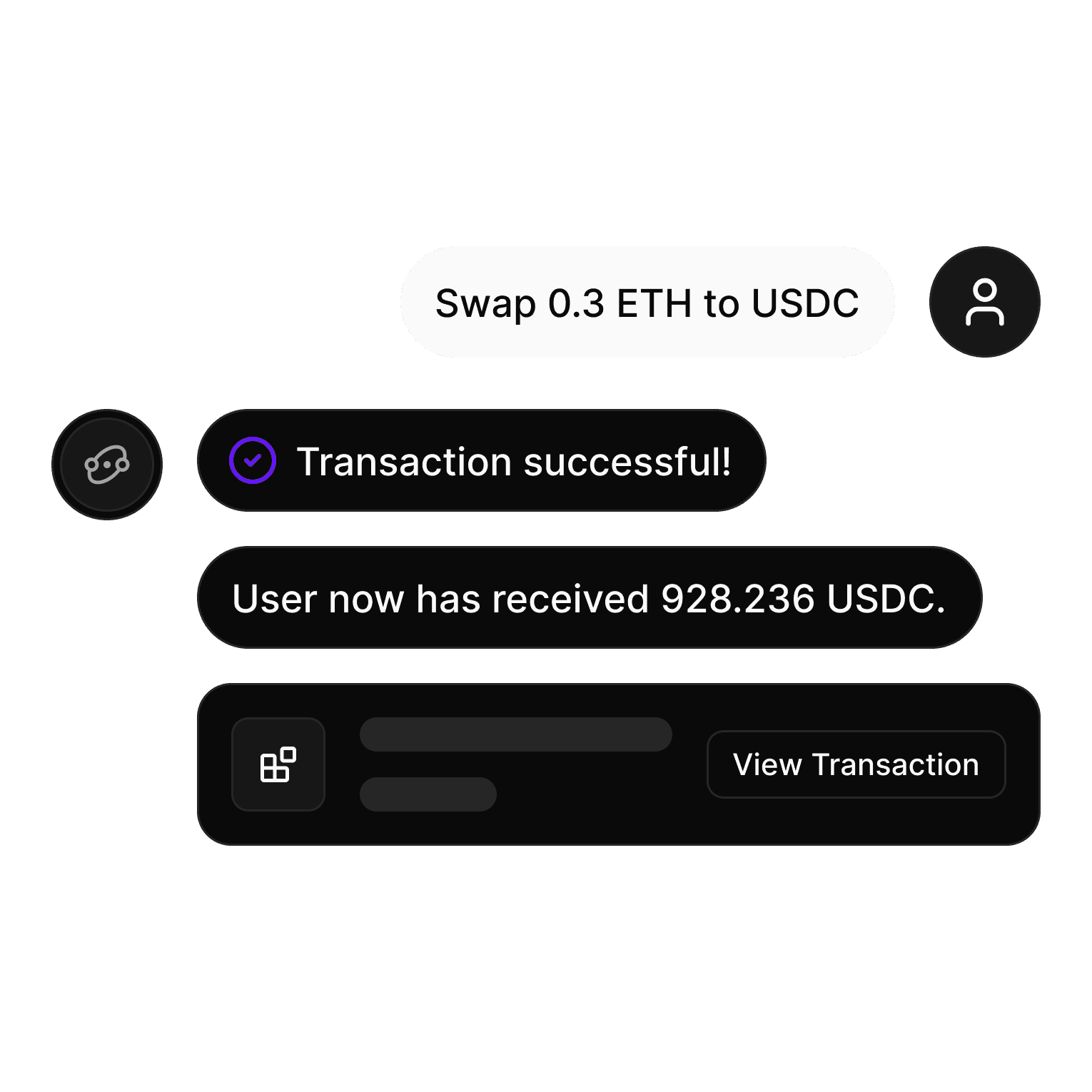
Insight
Simplify blockchain data queries
Use customizable endpoints to query rich blockchain data with flexibility — start from custom API schemas or use blueprints to source your data.
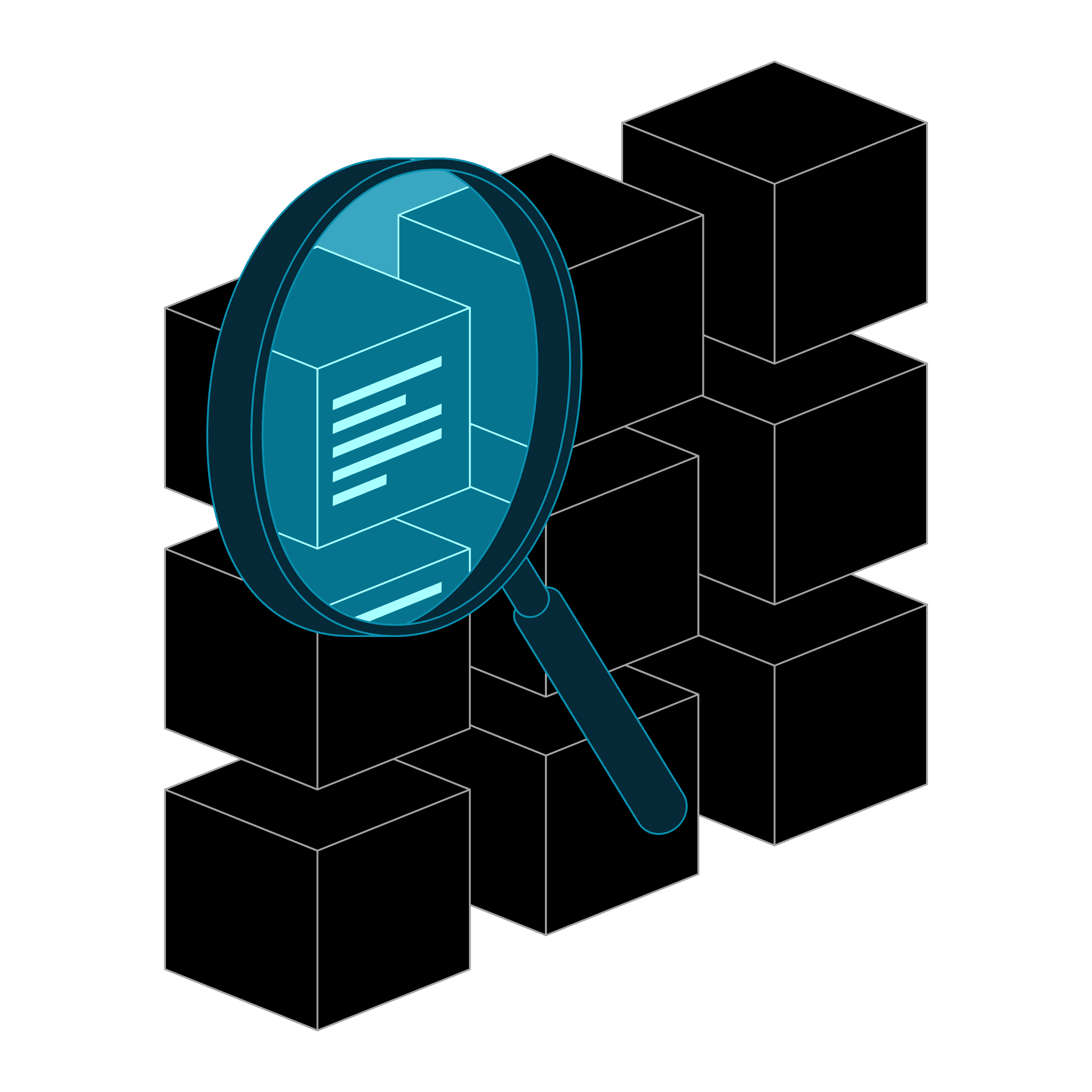
Insight
Simplify blockchain data queries
Use customizable endpoints to query rich blockchain data with flexibility — start from custom API schemas or use blueprints to source your data.
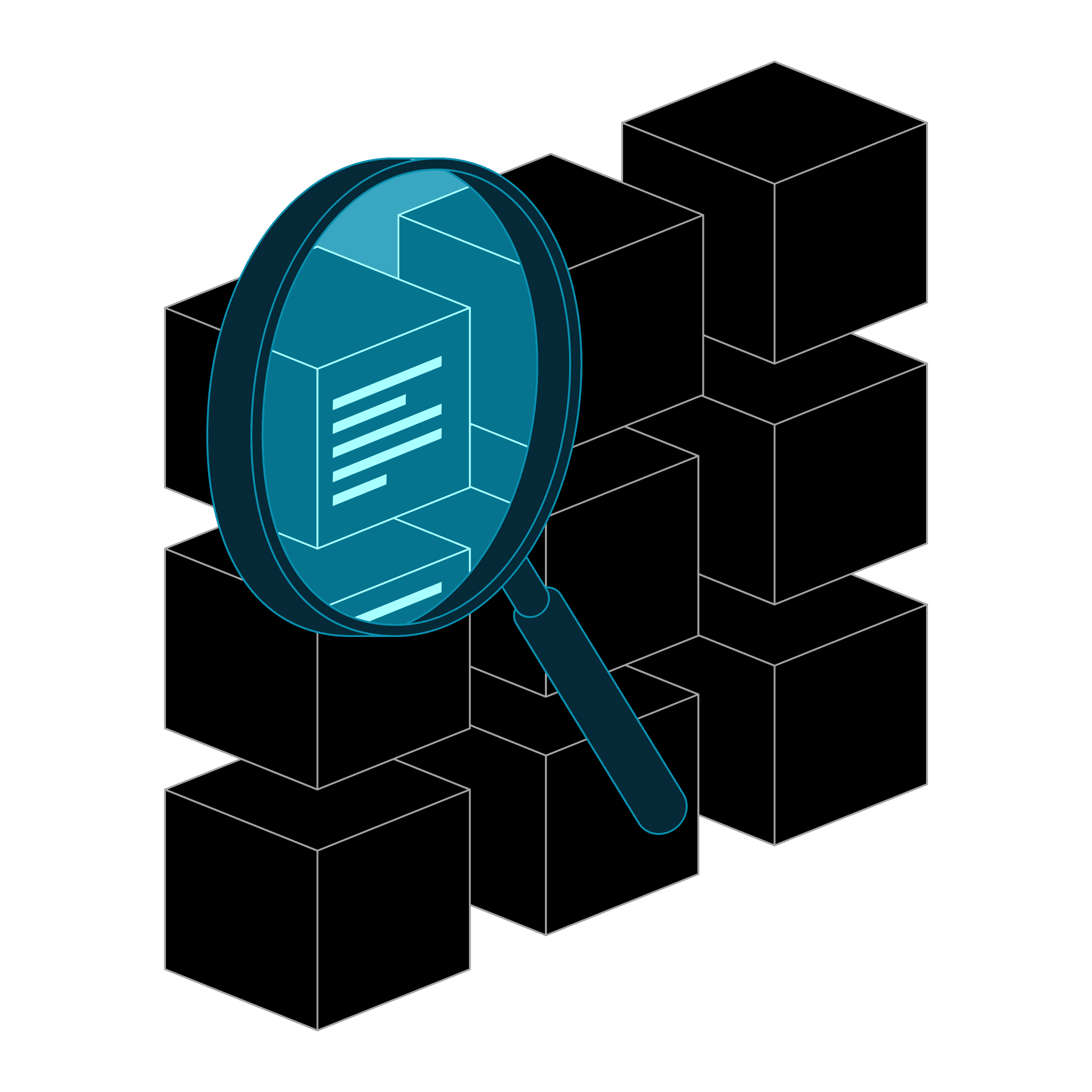
Insight
Simplify blockchain data queries
Use customizable endpoints to query rich blockchain data with flexibility — start from custom API schemas or use blueprints to source your data.
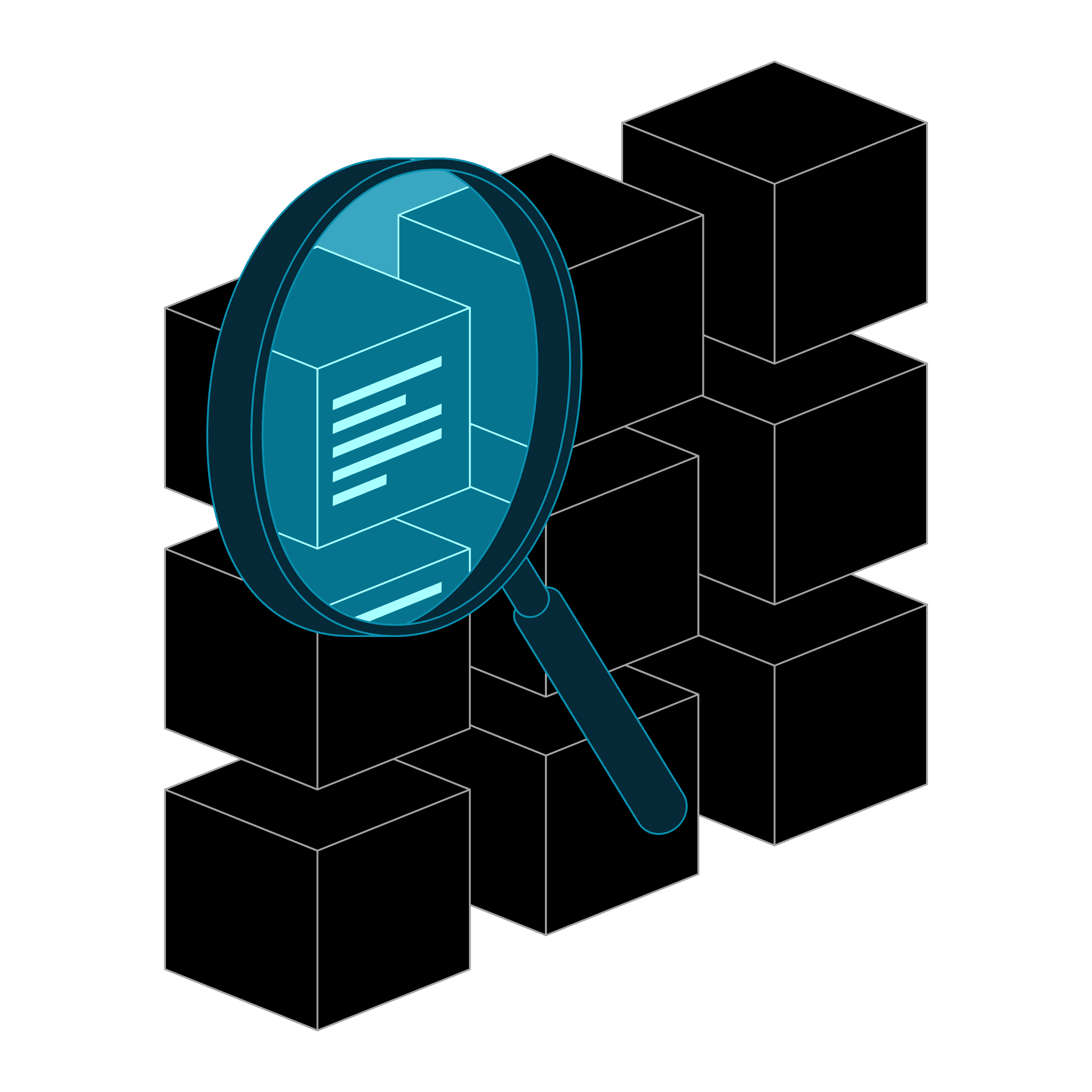
Connect
Client SDKs to connect users to web3
Onboard every user, connect to any crypto wallet, and enable social login — with in-app wallets, account abstraction, and fiat & crypto payments.
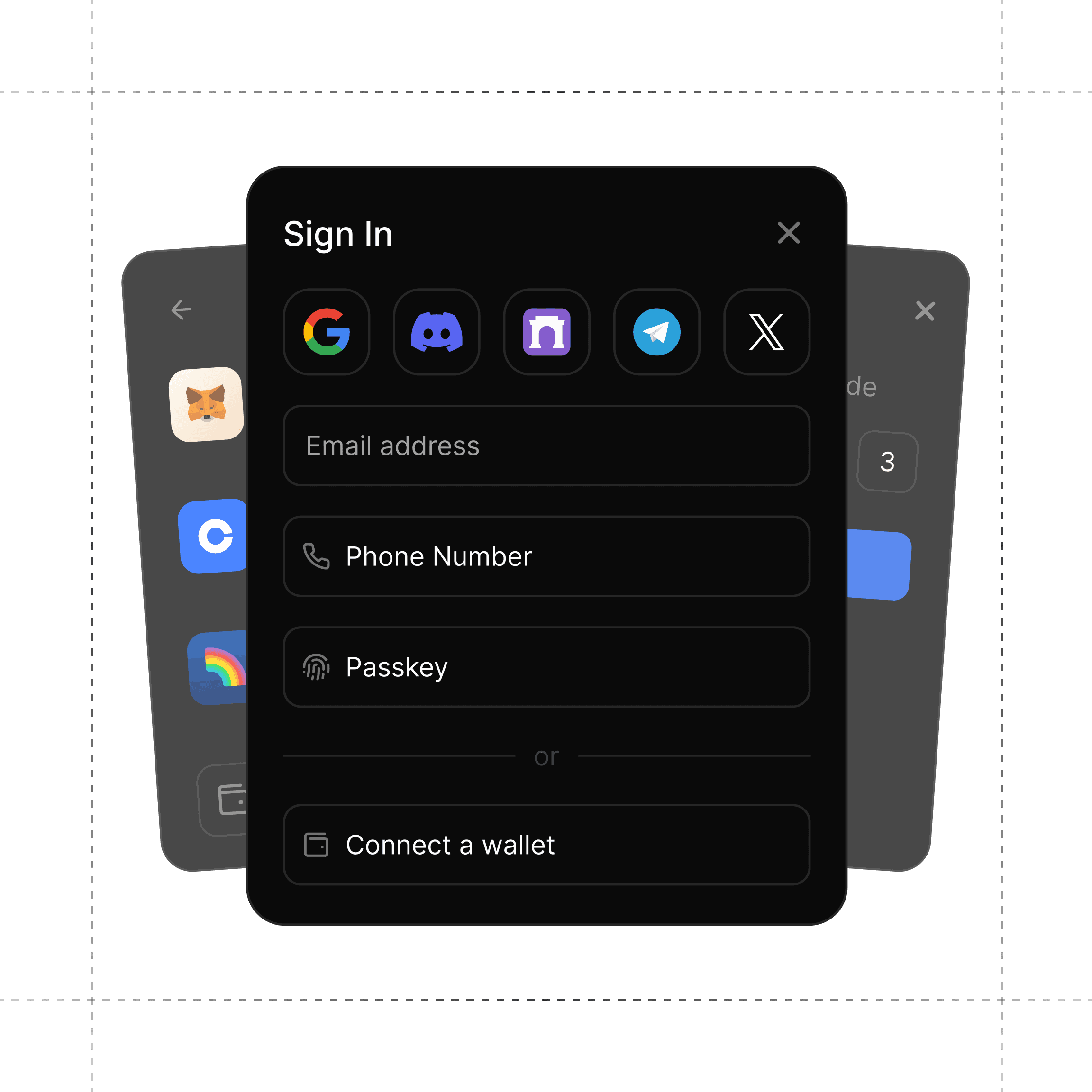
Connect
Client SDKs to connect users to web3
Onboard every user, connect to any crypto wallet, and enable social login — with in-app wallets, account abstraction, and fiat & crypto payments.
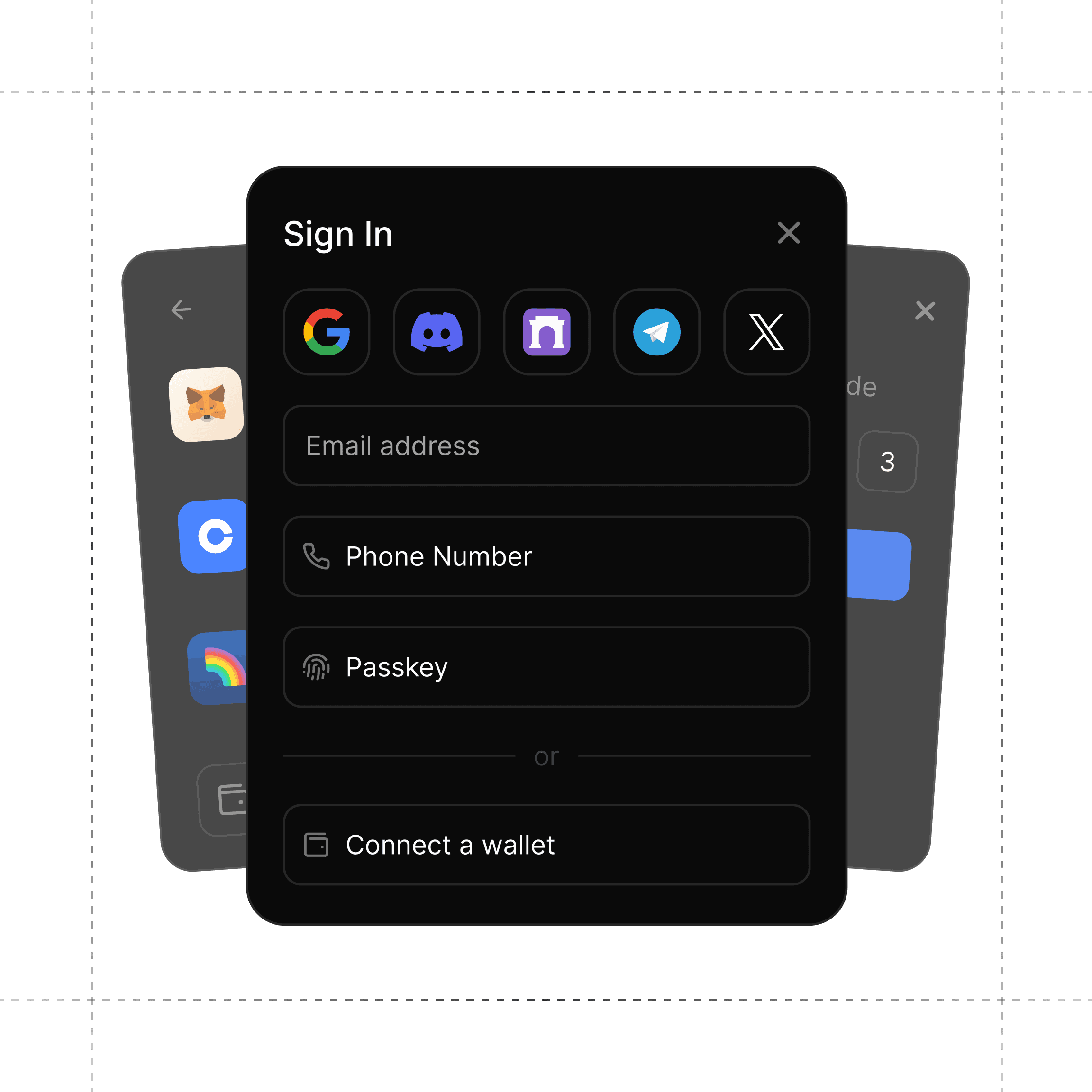
Connect
Client SDKs to connect users to web3
Onboard every user, connect to any crypto wallet, and enable social login — with in-app wallets, account abstraction, and fiat & crypto payments.
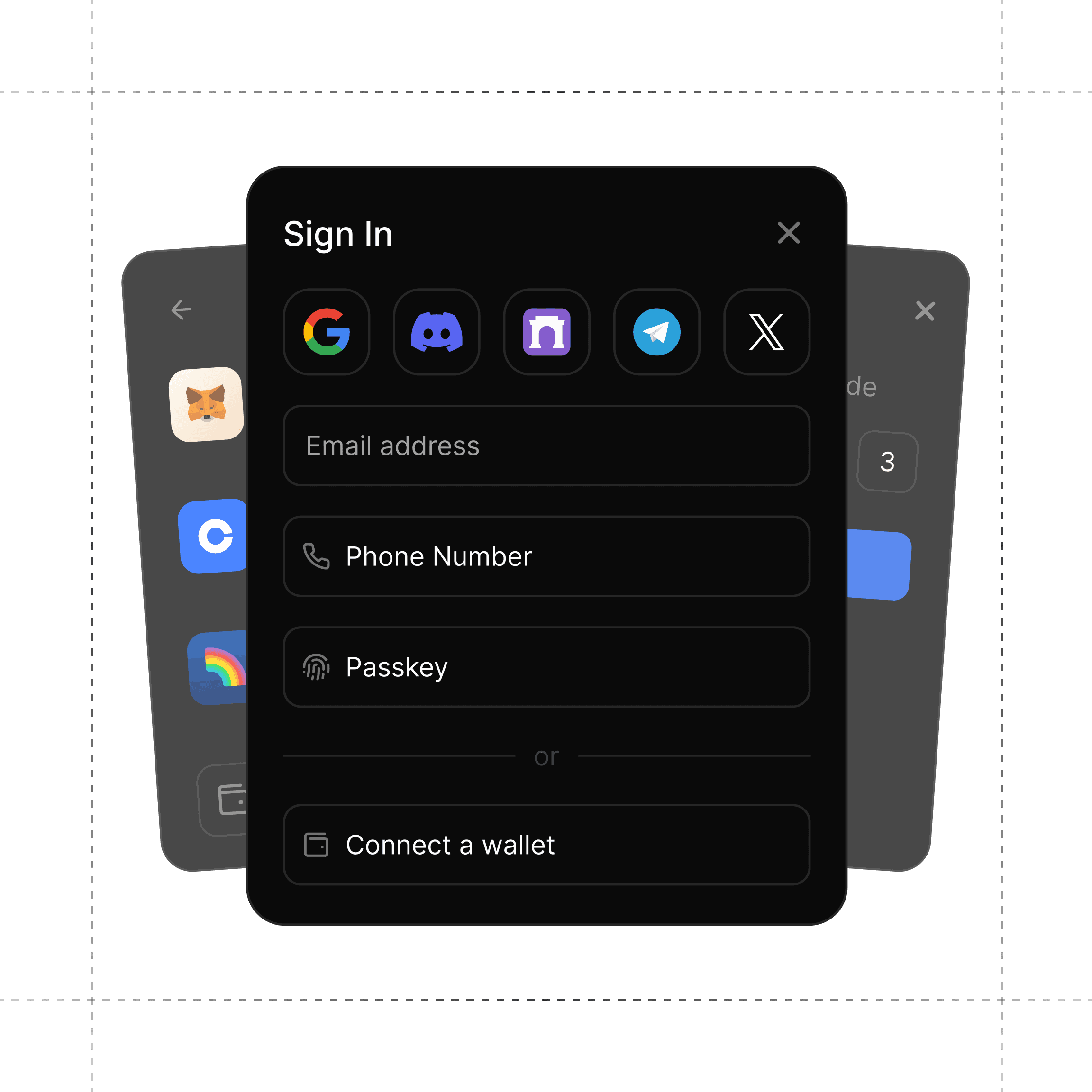
Contracts
End-to-end smart contract tools
Secure smart contract templates, an efficient modular smart contract framework & Solidity SDK, so you can deploy on EVM chain.
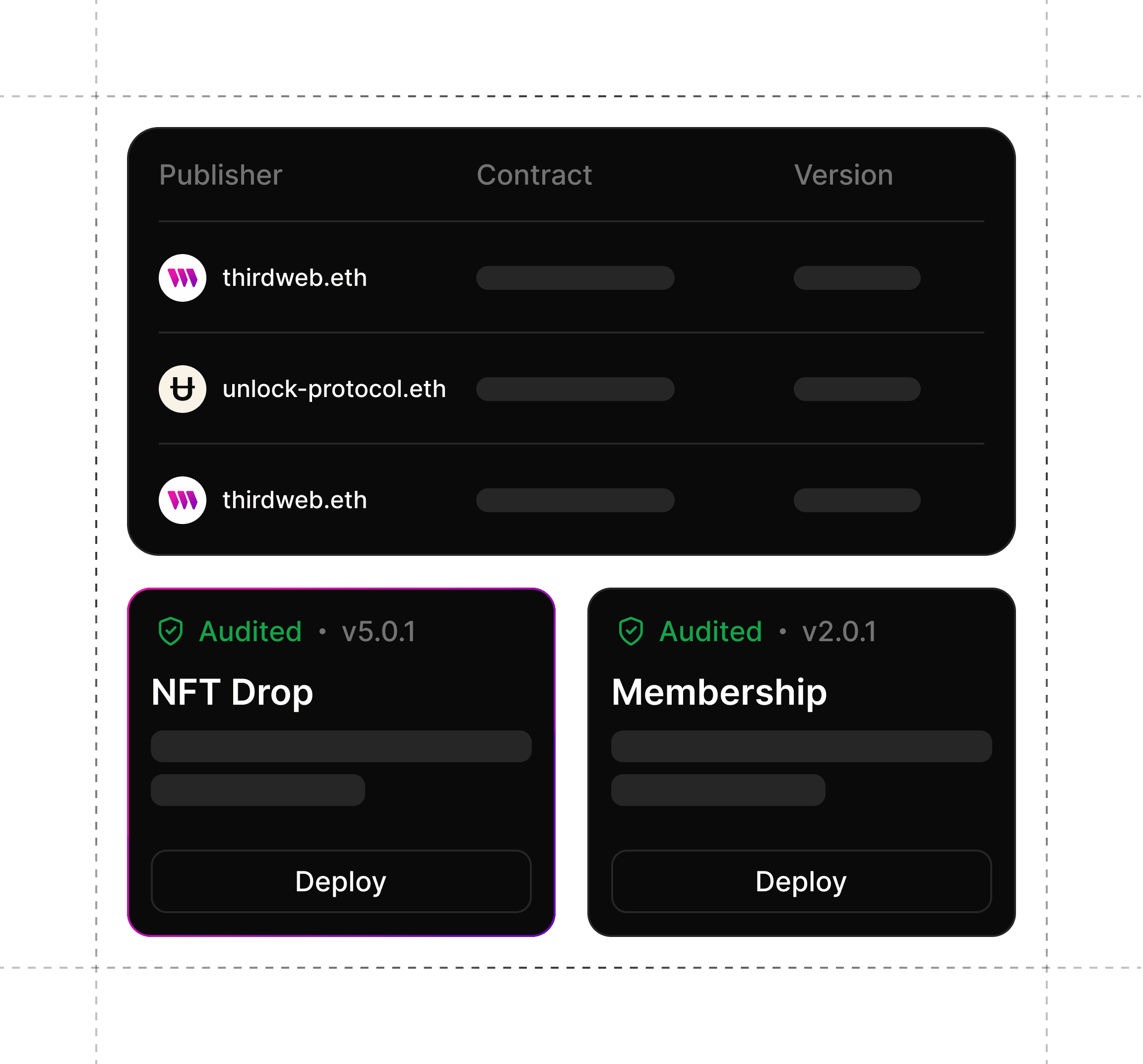
Contracts
End-to-end smart contract tools
Secure smart contract templates, an efficient modular smart contract framework & Solidity SDK, so you can deploy on EVM chain.
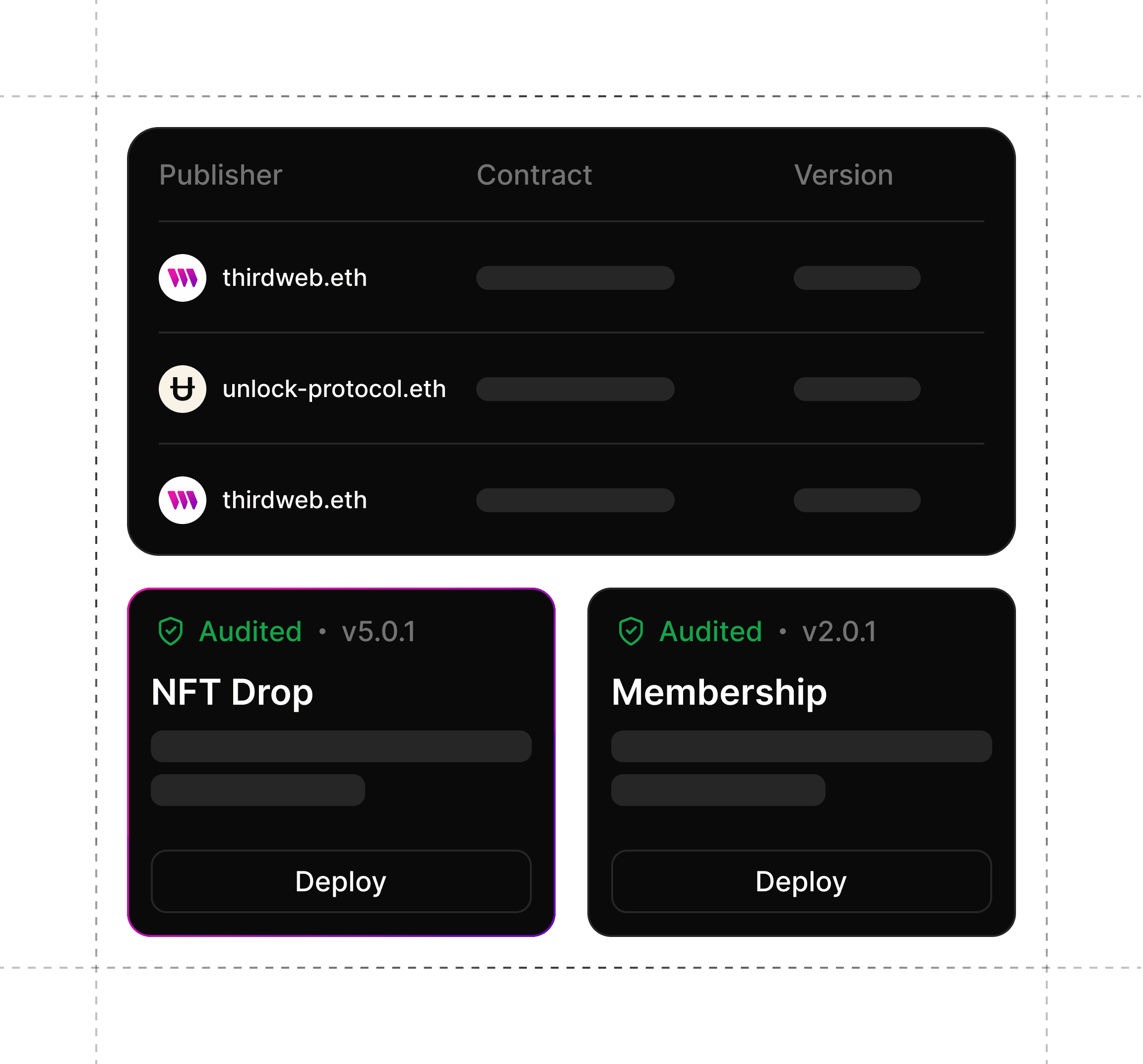
Contracts
End-to-end smart contract tools
Secure smart contract templates, an efficient modular smart contract framework & Solidity SDK, so you can deploy on EVM chain.
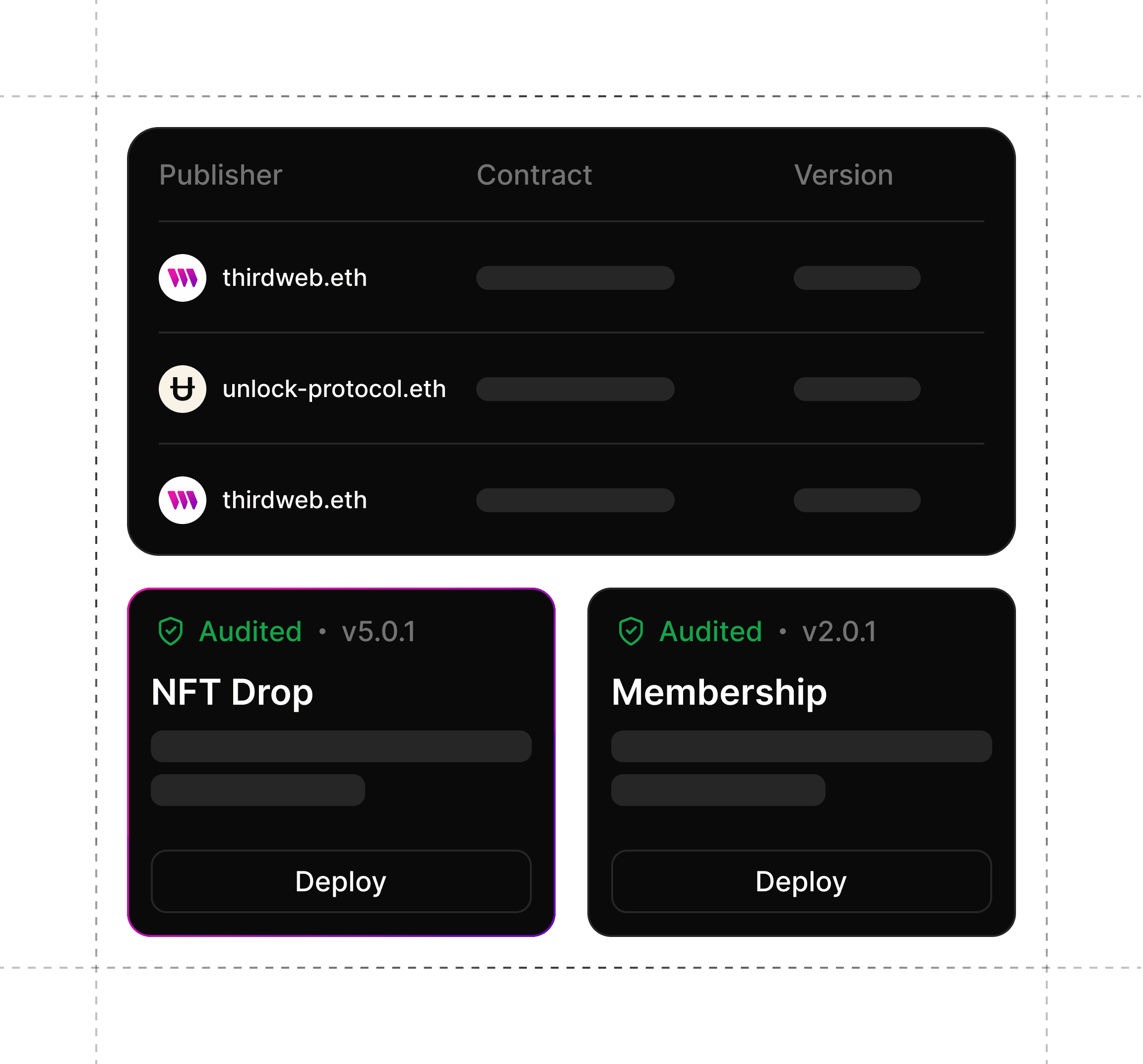
Engine
Dedicated APIs for web3 apps & games
Scalable blockchain APIs backed by secure wallets, with automatic nonce queuing & gas-optimized retries.
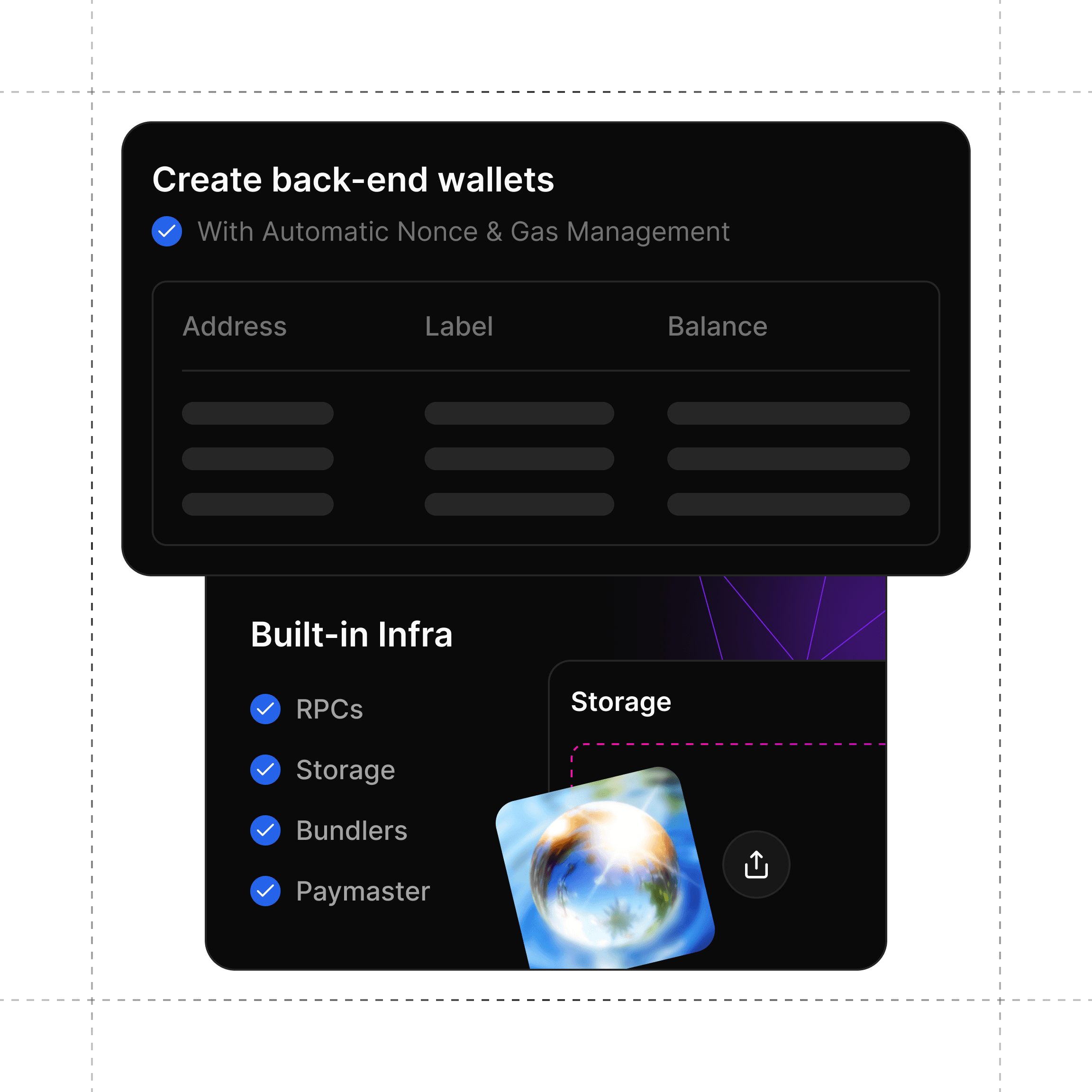
Engine
Dedicated APIs for web3 apps & games
Scalable blockchain APIs backed by secure wallets, with automatic nonce queuing & gas-optimized retries.
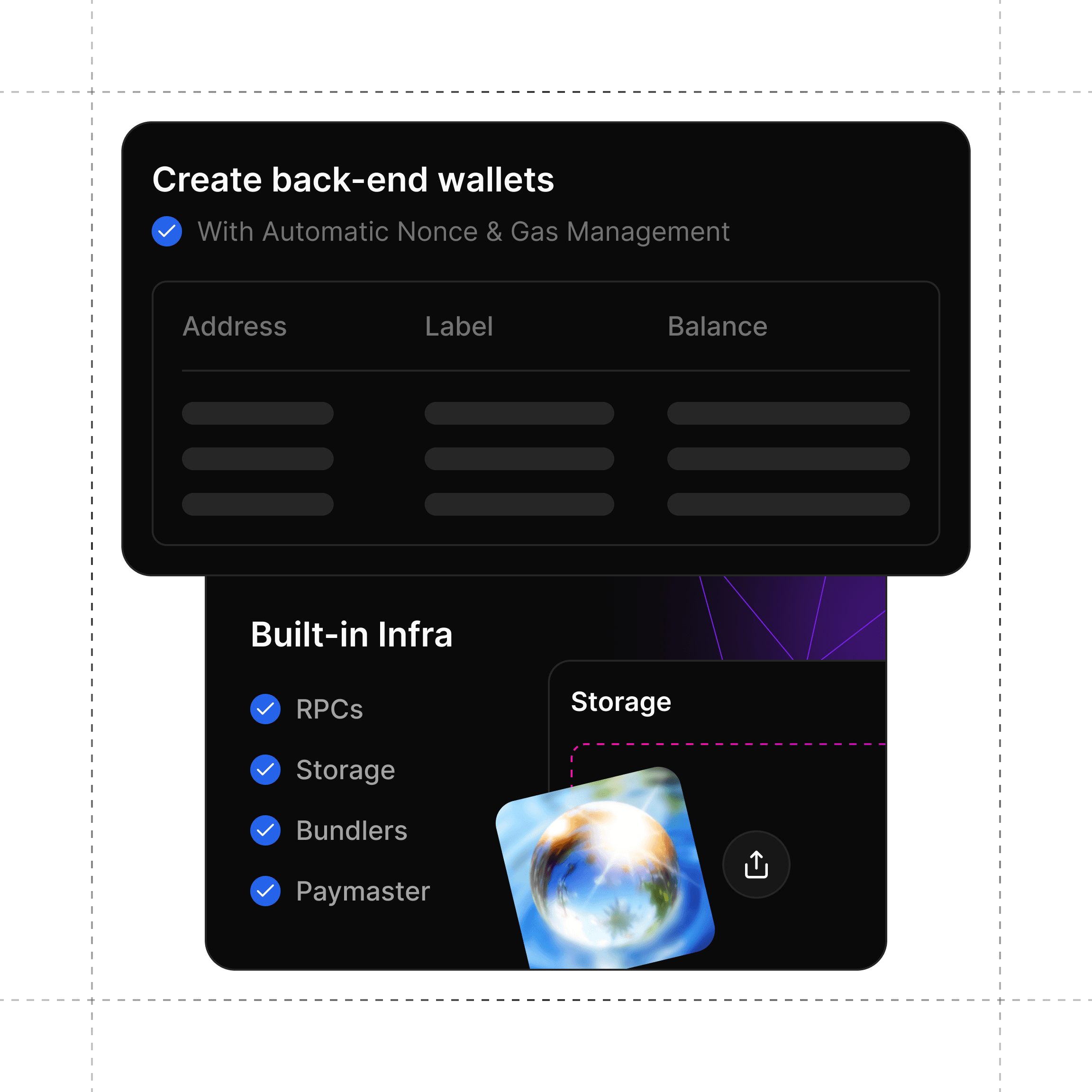
Engine
Dedicated APIs for web3 apps & games
Scalable blockchain APIs backed by secure wallets, with automatic nonce queuing & gas-optimized retries.
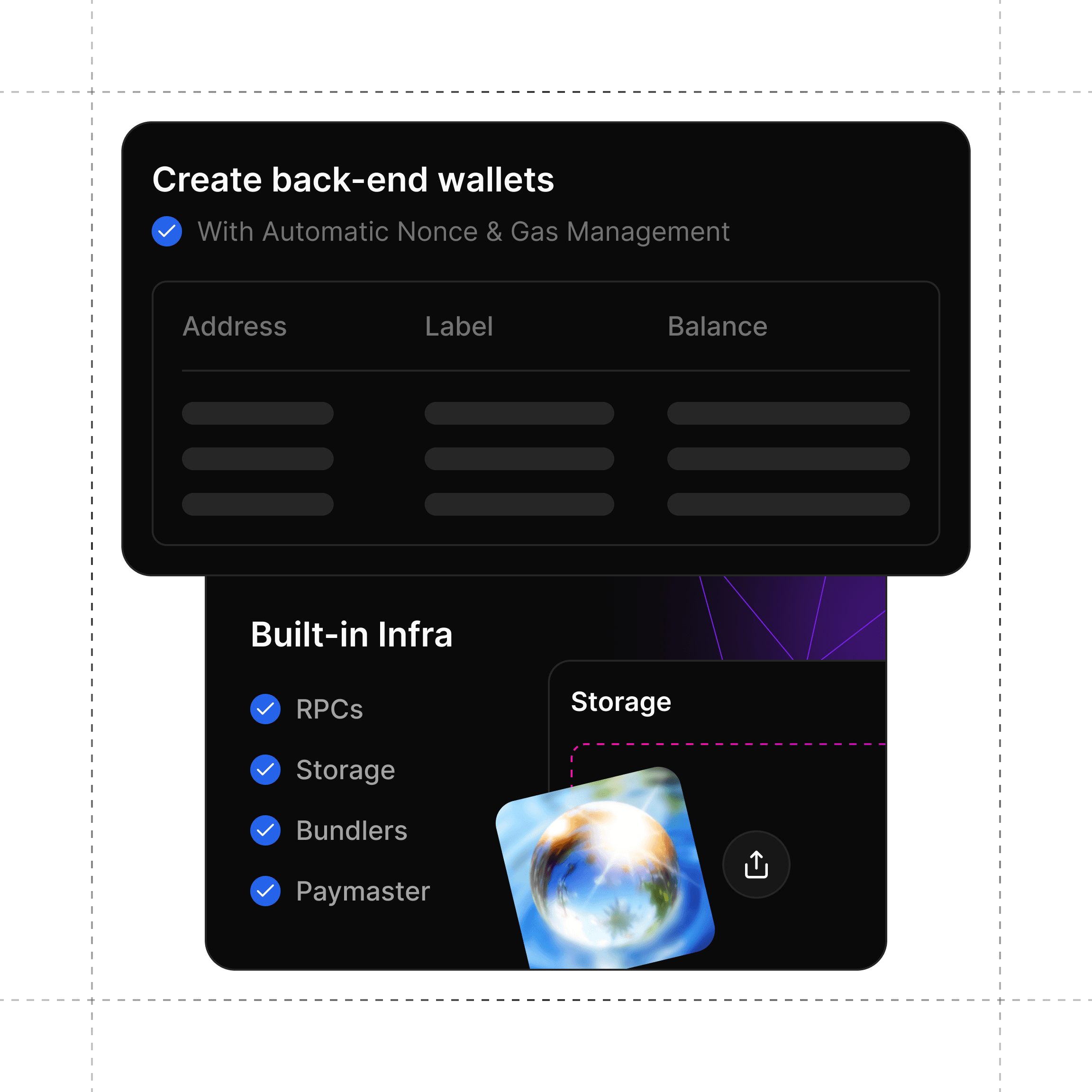
In any language on every platform.
Typescript
React
React Native
.NET
Unity
index.tsx
import { createThirdwebClient, getContract } from "thirdweb"; import { sepolia } from "thirdweb/chains"; // initialize the client const client = createThirdwebClient({ clientId }); // connect to your smart contract const contract = getContract({ client, chain: sepolia, address: "0x..." }); // get all NFTs const nfts = await getNFTs({ contract }); console.info(nfts);
In any language on every platform.
Typescript
React
React Native
.NET
Unity
index.tsx
import { createThirdwebClient, getContract } from "thirdweb"; import { sepolia } from "thirdweb/chains"; // initialize the client const client = createThirdwebClient({ clientId }); // connect to your smart contract const contract = getContract({ client, chain: sepolia, address: "0x..." }); // get all NFTs const nfts = await getNFTs({ contract }); console.info(nfts);
index.tsx
import { createThirdwebClient, getContract } from "thirdweb"; import { sepolia } from "thirdweb/chains"; // initialize the client const client = createThirdwebClient({ clientId }); // connect to your smart contract const contract = getContract({ client, chain: sepolia, address: "0x..." }); // get all NFTs const nfts = await getNFTs({ contract }); console.info(nfts);
In any language on every platform.
Trusted by the best
Powering web3 apps across verticals — from onchain games to creator platforms.
Trusted by the best
Powering web3 apps across verticals — from onchain games to creator platforms.
Trusted by the best
Powering web3 apps across verticals — from onchain games to creator platforms.
Build on any EVM chain
Our tools work with any contract deployed on any EVM-compatible chain.
Build on any EVM chain
Our tools work with any contract deployed on any EVM-compatible chain.
Build on any EVM chain
Our tools work with any contract deployed on any EVM-compatible chain.
Plans that scale to every team
Flexible and transparent pricing that scales to your app usage without cutting you off, ever.
Starter
Ideal for hobbyists who require basic features.
$9
/ month
$25 Usage Credits
Community Support
Web, Mobile & Gaming SDKs
Contract & Wallet APIs
Account Abstraction
Audited smart contracts
Blockchain infra (RPC, IPFS)
Engine Cloud
Growth
Popular Choice
Ideal for scalable production-grade apps.
$79
/ month
$100 Usage Credits
Email Support
Everything in Starter, plus:
SMS Onboarding
30 Day User Analytics
Gas grant for transactions
Custom Wallet Branding & Auth
Accelerate
For funded startups and mid-size businesses.
$249
/ month
$250 Usage Credits
Everything in Growth, plus:
24hr Guaranteed Response
90 Day User Analytics
Scale
For large organizations with custom needs.
$549
/ month
$500 Usage Credits
All-Time User Analytics
12hr Guaranteed Response
Slack Channel Support
Everything in Accelerate, plus:
Ultra-High Rate Limits
Need more? Upgrade to Pro
Custom configurations, full support, enterprise-grade solutions.
Plans that scale to every team
Flexible and transparent pricing that scales to your app usage without cutting you off, ever.
Starter
Ideal for hobbyists who require basic features.
$9
/ month
$25 Usage Credits
Community Support
Web, Mobile & Gaming SDKs
Contract & Wallet APIs
Account Abstraction
Audited smart contracts
Blockchain infra (RPC, IPFS)
Engine Cloud
Growth
Popular Choice
Ideal for scalable production-grade apps.
$79
/ month
$100 Usage Credits
Email Support
Everything in Starter, plus:
SMS Onboarding
30d User Analytics
Gas grant for transactions
Custom Wallet Branding & Auth
Accelerate
For funded startups and mid-size businesses.
$249
/ month
$250 Usage Credits
Everything in Growth, plus:
24hr Guaranteed Response
90d User Analytics
Scale
For large organizations with custom needs.
$549
/ month
$500 Usage Credits
All-Time User Analytics
12hr Guaranteed Response
Slack Channel Support
Everything in Accelerate, plus:
Ultra-High Rate Limits
Need more? Upgrade to Pro
Custom configurations, full support, enterprise-grade solutions.
Plans that scale to every team
Flexible and transparent pricing that scales to your app usage without cutting you off, ever.
Starter
Ideal for hobbyists who require basic features.
$9
/ month
$25 Usage Credits
Community Support
Web, Mobile & Gaming SDKs
Contract & Wallet APIs
Account Abstraction
Audited smart contracts
Blockchain infra (RPC, IPFS)
Engine Cloud
Growth
Popular Choice
Ideal for scalable production-grade apps.
$79
/ month
$100 Usage Credits
Email Support
Everything in Starter, plus:
SMS Onboarding
30d User Analytics
Gas grant for transactions
Custom Wallet Branding & Auth
Accelerate
For funded startups and mid-size businesses.
$249
/ month
$250 Usage Credits
Everything in Growth, plus:
24hr Guaranteed Response
90d User Analytics
Scale
For large organizations with custom needs.
$549
/ month
$500 Usage Credits
All-Time User Analytics
12hr Guaranteed Response
Slack Channel Support
Everything in Accelerate, plus:
Ultra-High Rate Limits
Need more? Upgrade to Pro.
Custom configurations, full support, enterprise-grade solutions.
Solutions for every web3 app
Solutions for every web3 app
Solutions for every web3 app
Sign-up for our newsletter.
Get the latest web3 development news and updates.
Sign-up for our newsletter.
Get the latest web3 development news and updates.
Sign-up for our newsletter.
Get the latest web3 development news and updates.
Start with thirdweb.
Build web3 apps with ease. Get instant access.
Start with thirdweb.
Build web3 apps with ease. Get instant access.
Start with thirdweb.
Build web3 apps with ease. Get instant access.
Products
Networks
Products
Networks
Products
Networks